Let's code a raytracer in C++
Project source code available at :
https://github.com/kobr4/RayZUnderstanding the raytracing process and being able to write a minimal raytracer can be something useful while working on real-time 3D graphics, as often, light are pre-backed via raytracing calculation for performance purpose.
I would add that raytracing can produce the near-perfect computer-generated picture that real-time rastering technique try vaguely to imitate. (shadows, reflections, complex lighting models like radiosity, ambient occlusion).
This is an attempt to write a simple raytracer that I called RayZ (fancy name isn't it ?).
1. Getting started
The basis principle of a raytracer is that a ray is cast from the output camera to the rendered screen, and for each ray, one has to check whether the ray collides with any object of the scene, and further, determining what color would the screen pixel be by knowing the collided object material and lighting.
The have something running quickly I would stick to sphere primitive, as determining collision between a vector issued from a point in space and a sphere is super easy.
By placing a round sphere right in front of a camera and state the a pixel is "white if touching the sphere black else" , we will have a perfect white disc : great...but a little lack of...volume !
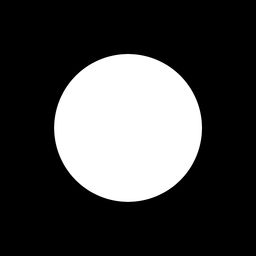
To add light we'll use Lambert lighting model(
http://en.wikipedia.org/wiki/Lambertian_reflectance ) : lighting is proportional to the angle made by the normal vector and light direction at contact point.
$\vec{N}$ : normal surface vector
$\vec{L}$ : normalized light direction vector
$I = \vec{N} \cdotp \vec{L}$
By adding a light source to our scene, here is the result :
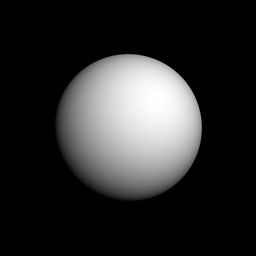
Pixel perfect shadow calculation is easy with raytracing : where the ray intersect sphere, we have to determine whether the line from the object to the lightsource intersects another object of the scene (and in that case, fallback to the ambient lighting).
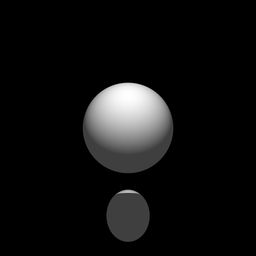
Coming next :
* Objects overlapping resolution using z-buffer
* Proper object rotation / translation transformations
* Proper color / material /lighting calculation and rendering
* Triangle rendering
* Simple scene description language